HTML/Document Trick
Contents
Understanding offsetWidth, clientWidth, scrollWidth
Note
All these property will round the value to an integer. If you need a fractional value, use element.getBoundingClientRect().
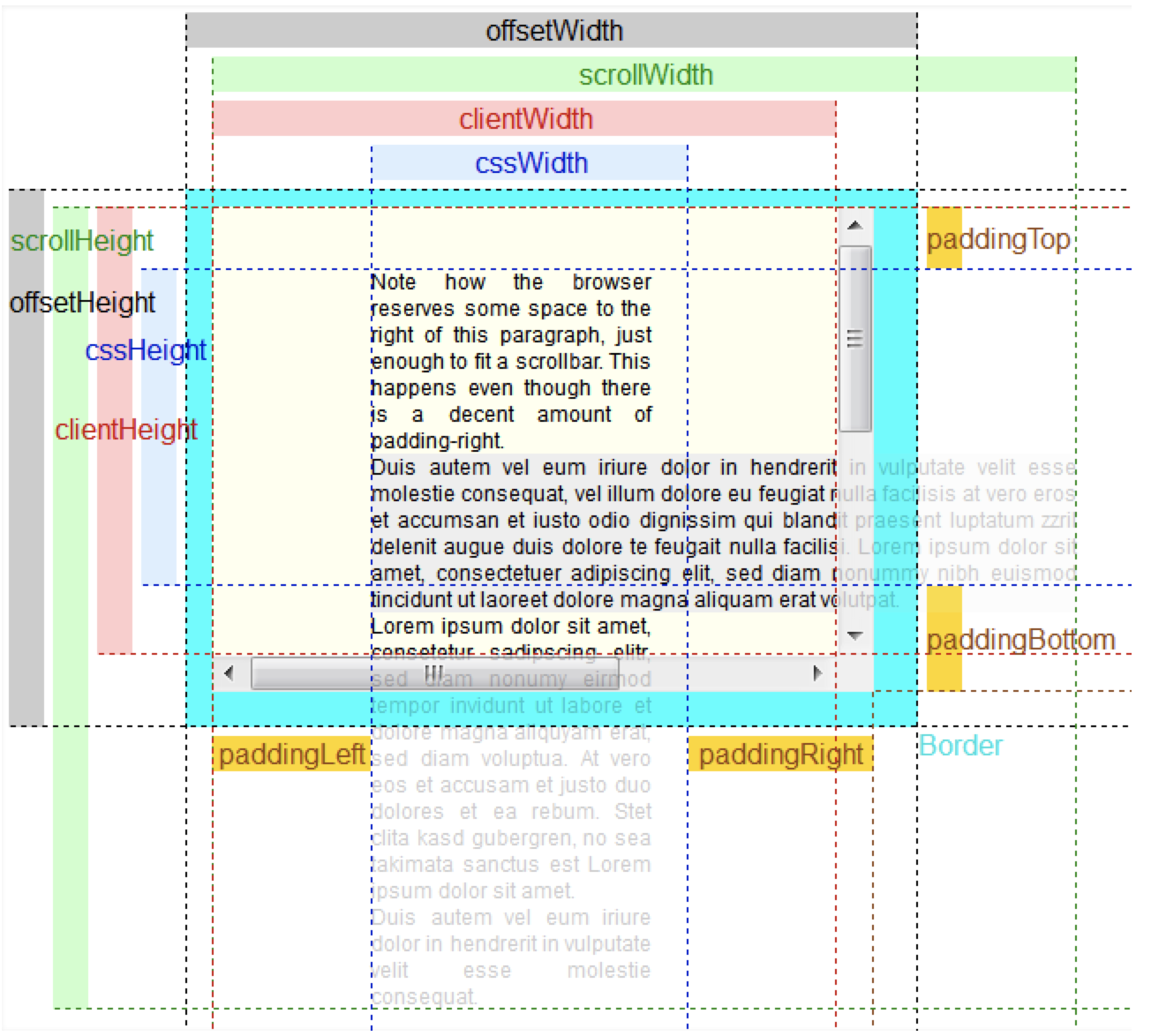
Get Scrollbar width
Reference: https://www.30secondsofcode.org/js/s/get-scrollbar-width/
Get window scroll bar width
const getScrollbarWidth = () =>
window.innerWidth - document.documentElement.clientWidth;
getScrollbarWidth();
Get a element scroll bar width
const getScrollbarWidth = (el) => {
const leftBorder = parseInt(
getComputedStyle(el).getPropertyValue('border-left-width'),
10
);
const rightBorder = parseInt(
getComputedStyle(el).getPropertyValue('border-right-width'),
10
);
return el.offsetWidth - el.clientWidth - leftBorder - rightBorder;
};
getScrollbarWidth(el);
Element: getBoundingClientRect()
el.getBoundingClientRect()
will return: left
, top
, right
, bottom
, x
, y
, width
, and height
.
x
===left
&y
===top
- In the standard box model,
width
andheight
will automatically calculatepadding
andborder-width
.- if
box-sizing: border-box
is set for the element this would be directly equal to itswidth
orheight
.
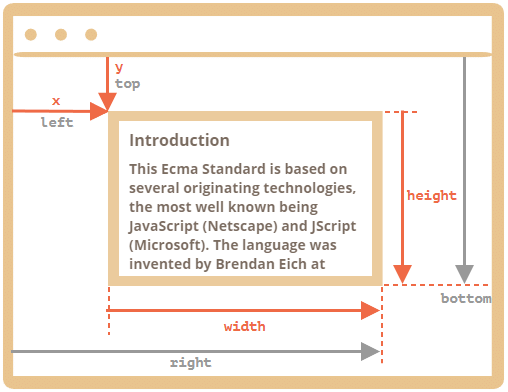